Introduction
Rust is a modern systems programming language that offers strong safety guarantees while providing high performance. One of the key aspects of Rust is its strong type system, which ensures memory safety without sacrificing performance. In this blog post, we'll delve into how Rust handles strings and integers, two fundamental data types in programming, and explore some of the unique features and capabilities Rust offers in this regard.
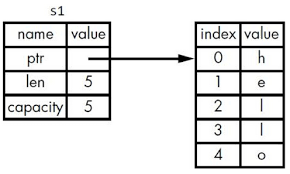
Strings in Rust
In Rust, strings are represented by the String type, which is a growable, mutable, UTF-8 encoded string. Rust's approach to strings is quite different from languages like C or C++, where strings are represented as arrays of characters.
""""
fn main() {
let greeting = String::from("Hello, world!");
println!("{}", greeting);
}
In the above example, we create a new String object called greeting and initialize it with the text "Hello, world!". We then print the string using println! macro.
Example
""""
fn main() {
let s = String::from("hello");
let slice = &s[0..2]; // slice will be "he"
println!("{}", slice);
}
This feature allows for efficient string manipulation without unnecessary memory allocations. Rust also offers a variety of methods for working with strings, such as push_str, pop, replace, trim, etc., making it easy to perform common string operations.
Integers in Rust
Rust provides a rich set of integer types, each with its own specific size and behavior. Here are some of the integer types available in Rust:
i8, i16, i32, i64, i128:
Signed integers with 8, 16, 32, 64, and 128 bits respectively.
u8, u16, u32, u64, u128:
Unsigned integers with 8, 16, 32, 64, and 128 bits respectively.
isize and usize: Signed and unsigned integers with the size of a pointer on the target architecture.
Example
""""
fn main() {
let decimal = 98_222;
let hex = 0x_dead_beef;
let octal = 0o77;
let binary = 0b1111_0000;
}
Conclusion
Rust's approach to strings and integers reflects its design principles of safety, performance, and expressiveness. By providing powerful abstractions and compile-time checks, Rust empowers developers to write efficient and safe code while avoiding common pitfalls associated with these fundamental data types. Whether you're building systems software, web applications, or anything in between, Rust's robust support for strings and integers makes it a compelling choice for a wide range of programming tasks.